Typescript
Best Practices for Typescript with React Native 2024
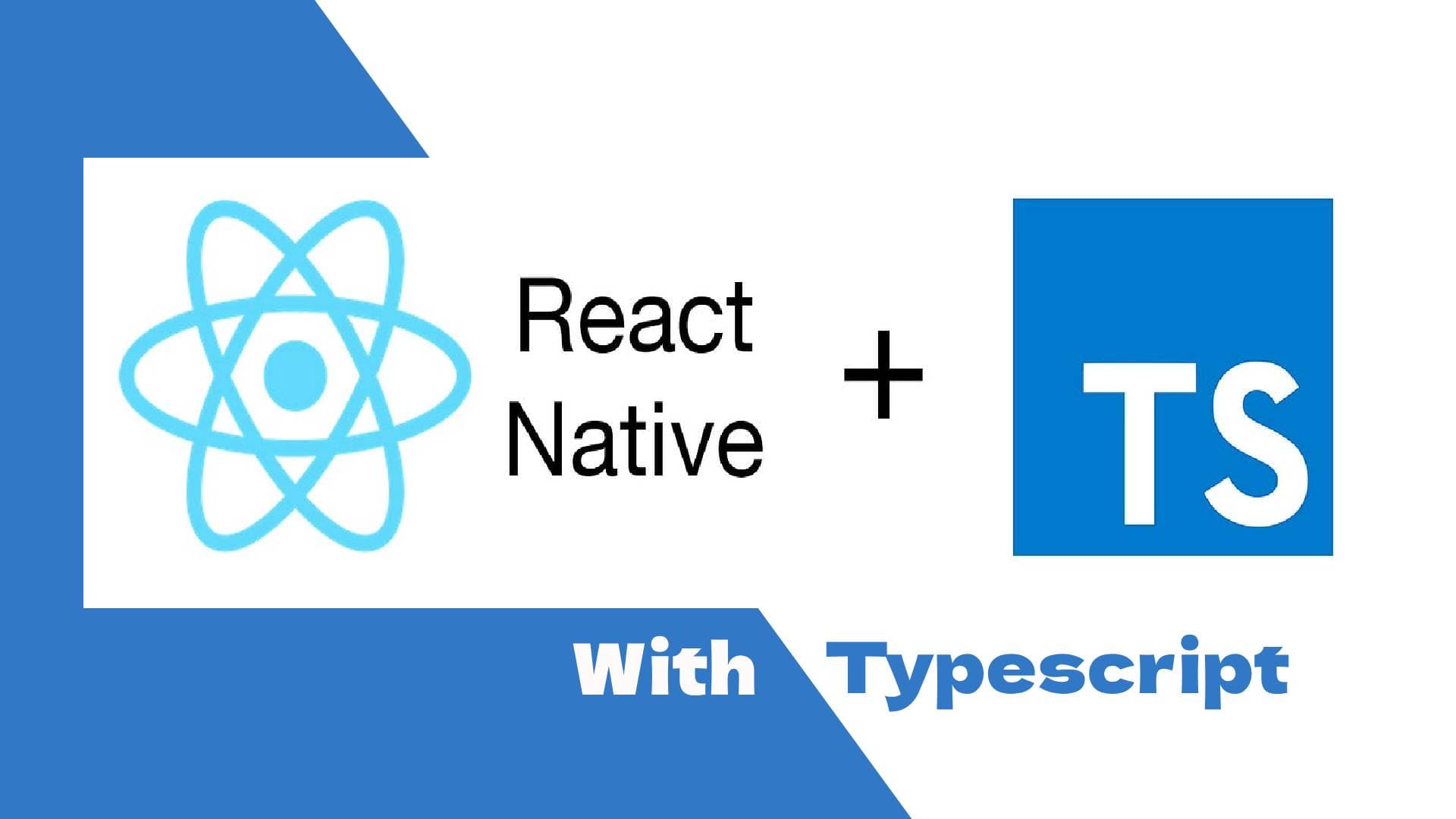
Table Of Content
Essential TypeScript Features for React Native Development
TypeScript has become a fundamental tool in React Native development, offering enhanced type safety and improved developer experience. This guide covers crucial TypeScript features for building React Native applications.
Key TypeScript Features for React Native
- Typing Component Props
type Props = {
title: string;
onPress?: () => void;
style?: StyleProp<ViewStyle>;
children: React.ReactNode;
};
const CustomComponent: React.FC<Props> = ({ title, onPress, style, children }) => {
return (
<View style={[styles.container, style]}>
<Text>{title}</Text>
{children}
</View>
);
};
- Styling with TypeScript
import { StyleSheet, ViewStyle, TextStyle } from 'react-native';
interface Styles {
container: ViewStyle;
text: TextStyle;
}
const styles = StyleSheet.create<Styles>({
container: {
flex: 1,
padding: 20,
},
text: {
fontSize: 16,
color: '#000',
},
});
- Navigation Typing
type RootStackParamList = {
Home: undefined;
Profile: { userId: string };
Settings: undefined;
};
type Props = NativeStackScreenProps<RootStackParamList, 'Profile'>;
const ProfileScreen = ({ route, navigation }: Props) => {
const { userId } = route.params;
// Component logic
};
Best Practices
- Use Strict TypeScript Configuration
{
"compilerOptions": {
"strict": true,
"target": "esi2017",
"jsx": "react-native",
"noEmit": true,
"moduleResolution": "node"
}
}
- Type API Responses
interface User {
id: string;
name: string;
email: string;
}
const fetchUser = async (id: string): Promise<User> => {
const response = await fetch(`/api/users/${id}`);
return response.json();
};
- State Management with TypeScript
interface AppState {
isLoading: boolean;
user: User | null;
error: string | null;
}
const initialState: AppState = {
isLoading: false,
user: null,
error: null,
};
Advanced Patterns
- Generic Components
type ListProps<T> = {
data: T[];
renderItem: (item: T) => React.ReactElement;
keyExtractor: (item: T) => string;
};
function List<T>({ data, renderItem, keyExtractor }: ListProps<T>) {
return (
<FlatList
data={data}
renderItem={({ item }) => renderItem(item)}
keyExtractor={keyExtractor}
/>
);
}
- Custom Hooks with TypeScript
function useToggle(initialState: boolean = false): [boolean, () => void] {
const [state, setState] = useState(initialState);
const toggle = useCallback(() => setState(state => !state), []);
return [state, toggle];
}
Conclusion
Implementing TypeScript in React Native projects brings numerous benefits:
- Enhanced code reliability
- Better developer experience
- Improved maintainability
- Easier refactoring
Key takeaways:
- Always define proper types for components and functions
- Utilize React Native's built-in types
- Maintain consistent typing conventions
- Keep TypeScript configurations up to date
By following these practices, you'll create more robust and maintainable React Native applications.